Triangulation of convex polygons
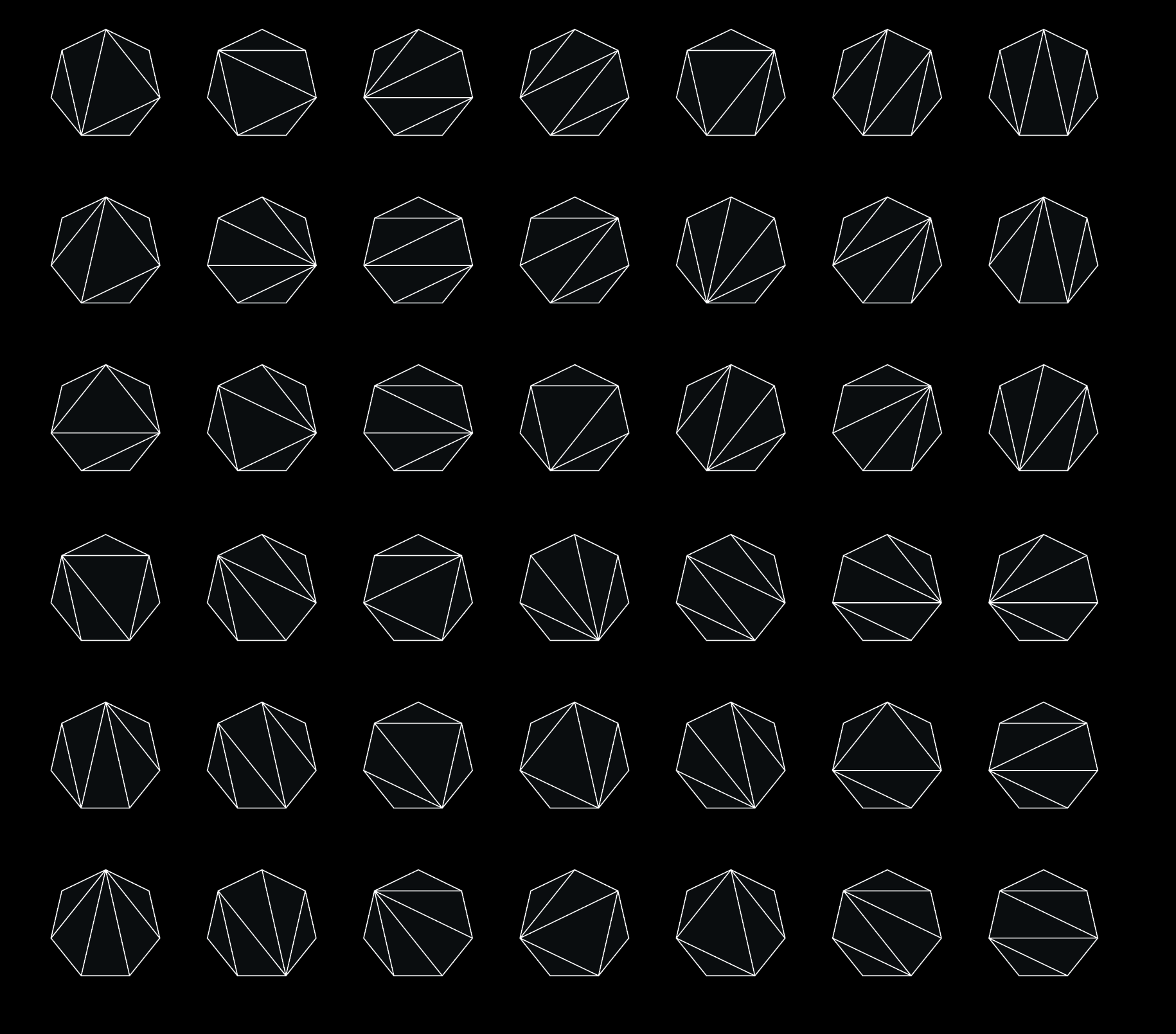
In this brief article, I will discuss the preliminaries of polygon triangulation using the APL programming language. Triangulation is an important concept in computational geometry, and it has applications in various fields, such as computer graphics, mesh generation, and finite element analysis. Triangular meshes are often used to represent surfaces in computer graphics, and they are also used in finite element analysis to approximate the solution of partial differential equations.
Introduction⌗
A convex polygon is a polygon in which all its interior angles are less than 180 degrees with vertices defined by , where is the number of vertices. Triangulation is the partitioning of a polygon with vertices into triangles by adding non-intersecting diagonals, which connect non-adjacent vertices.
Due to Euler, the total amount of ways to triangulate a -gon is given by the -th Catalan number:
Asymptotically, Catalan numbers grow as:
Consequently, the algorithm enumerating all possible triangulations of a polygon is exponential in time complexity.
Unconstrainted triangulation⌗
If we wish to triangulate a polygon without any constraints, the fan triangulation of any polynomial can be computed in linear time:
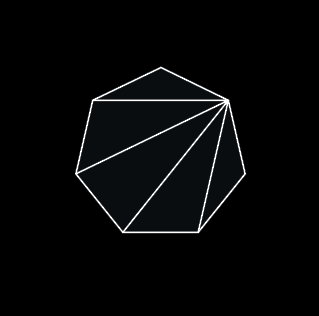
Once we pick a starting vertex , we can connect it to all other vertices , creating triangles.
Another method of unconstrained triangulation is the ear-clipping algorithm, which is computed in time. The algorithm iteratively removes “ears” from the polygon, where an ear is a triangle with two consecutive edges of the polygon and the third edge inside the polygon. We begin the implementation with a function that given three points determines if they form a convex angle:
The next step is to determine, given a point , whether it lies inside the triangle . For this purpose, we could make use of barycentric coordinates, but the naive method (checking on which side of the half-plane created by the edges the point resides) is sufficient for our purposes:
The method for finding the ears is then straightforward. We return the ear and the rest of the polygon in a tuple. We implement checks in order to determine if the polygon is large enough, then iterate over the consecutive 3-triplets of vertices, to then determine if they form an ear. The first ear found is returned and its vertex is removed from the polygon.
The triangulation function successively removes ears from the polygon until only three vertices remain and returns the resulting triangulation:
To test our code, we will use the following polygon:
We will also plot it using Desmos:
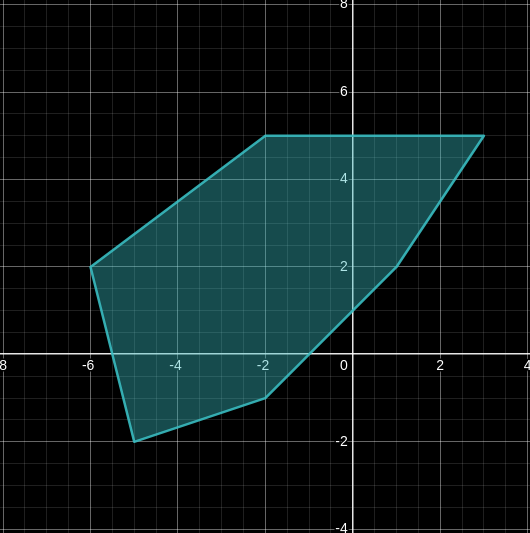
The resulting triangulation is given by:
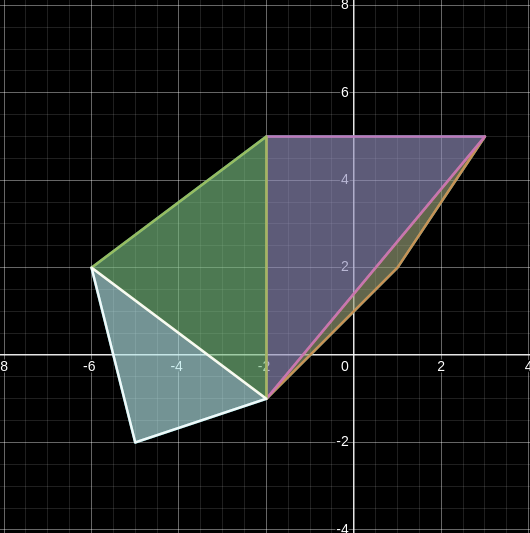
Constrained triangulation⌗
Suppose that we wish to triangulate a -gon into triangles , where each triangle is defined by the vertices . We can then define a cost function that assigns a cost to each triangle. The cost function can be defined in various ways, such as the area of the triangle, the length of the edges, or the angle between the edges. The constrained triangulation problem is then to find the cost of the triangulation that minimizes the cost function.
Suppose that we have the following cost function, which assigns the cost of a triangle to be the sum of the lengths of its edges:
The naive solution to this problem runs in exponential time through testing all possible triangulations:
Nonetheless, for small polygons, it’s rather fast:
We can improve upon this solution making use of Dynamic Programming: Many recursive calls in the naive solution are repeated, so we can store the results of these calls in a table and use them to avoid recomputation. We accomplish it via the use of the memo
operator from the dfns
workspace: